This post includes an explanation of the importance of React in the workplace and includes 50 interview questions about React.
Faster job search. More Offers.
Use our AI Cover Letter Builder, Interview Prep and Job Search Tools to land your next job.
What is React?
React is a popular, open-source JavaScript library used for building user interfaces, particularly for single-page applications. It allows developers to create large web applications that can change data, without reloading the page. Every significant application built with React is made up of multiple components, each responsible for outputting a small, reusable piece of HTML code. The key advantage of React is its speed, scalability, and simplicity. It also has a strong supporting community and is maintained by Facebook. If you're looking into enhancing your technical skill set, particularly in front-end development, learning React could be a valuable asset.
Why is React important in the workplace?
1. Adaptability in a Fast-Paced Environment
The ability to react swiftly and effectively in the workplace is crucial in today's fast-paced business environment. This skill ensures that employees can handle sudden changes, challenges, or opportunities with ease, maintaining productivity and preventing disruptions.
2. Enhanced Problem-Solving
Reacting appropriately to unforeseen issues or obstacles is a testament to an employee's problem-solving skills. It involves quickly understanding the problem, assessing possible solutions, and implementing the most effective one. This capability not only minimizes the impact of issues but also contributes to continuous improvement within the organization.
3. Improved Communication and Collaboration
Effective reaction skills are not just about responding to situations but also about how one communicates and collaborates with others during these times. Being able to react promptly and constructively in discussions, meetings, or team projects fosters a more dynamic and cohesive work environment, encouraging teamwork and enhancing overall productivity.
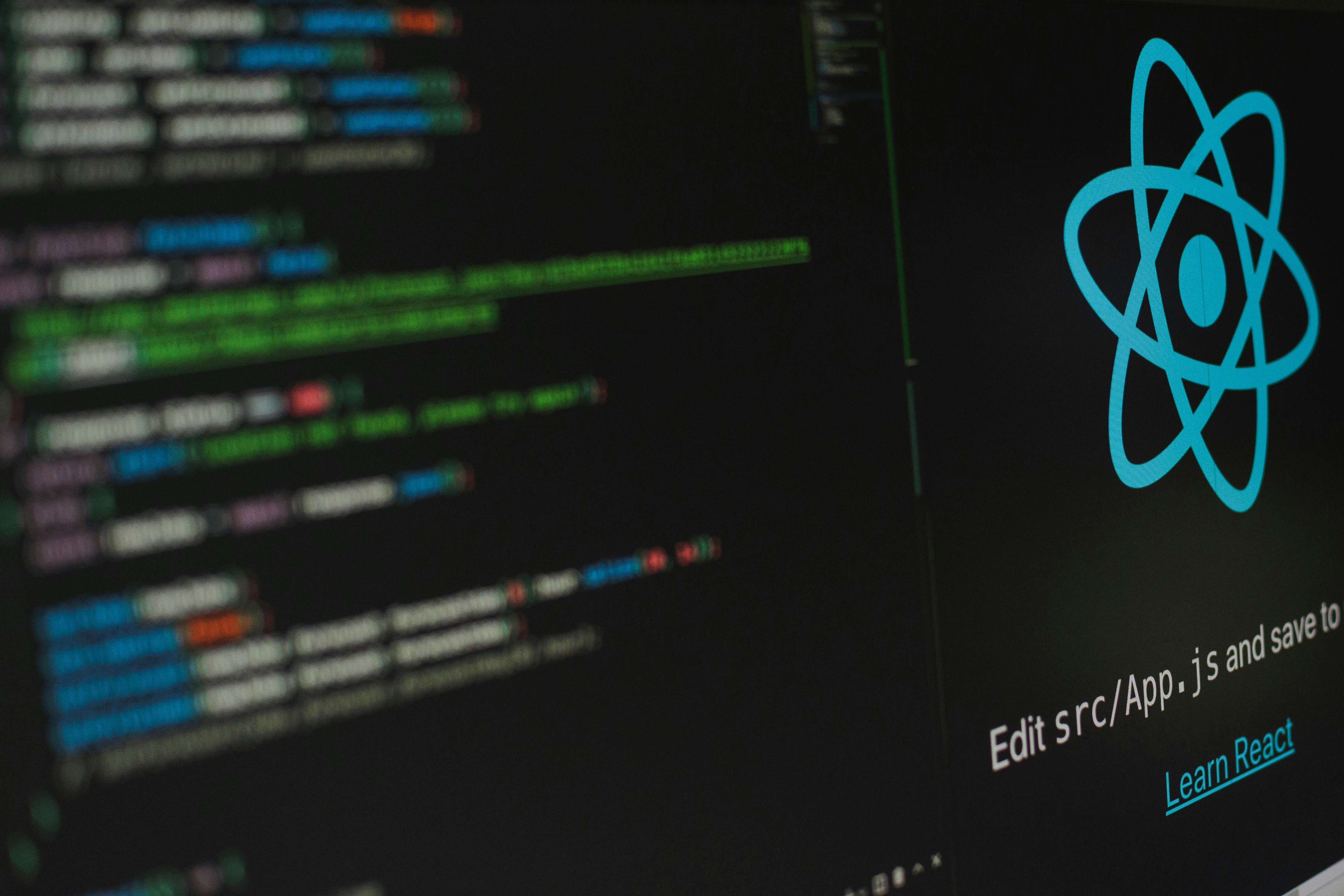
50 Interview Questions About React (With Answers)
1. Can you explain what React is and why it's used in web development?
React is a JavaScript library developed by Facebook for building user interfaces, particularly for single-page applications. It allows developers to create reusable UI components, which can manage their own state and be composed to build complex UIs. React is known for its efficiency and flexibility, thanks to its use of the Virtual DOM and its component-based architecture. It simplifies the process of building interactive and dynamic user interfaces, making it a popular choice for web development.
2. How does React differ from other JavaScript frameworks like Angular or Vue.js?
React is often compared to frameworks like Angular and Vue.js, but there are key differences. React is a library focused solely on the view layer of an application, whereas Angular is a full-fledged MVC framework that includes everything from routing to state management. Vue.js, like React, is more focused on the view layer but offers more built-in features than React. React’s use of JSX allows developers to write HTML-like syntax directly in JavaScript, which is different from Angular's template system or Vue's SFCs (Single File Components). Additionally, React's Virtual DOM offers a performance edge by efficiently updating the UI without needing to re-render the whole page.
3. What is JSX and why is it important in React?
JSX stands for JavaScript XML. It is a syntax extension for JavaScript that allows developers to write HTML-like code within their JavaScript files. This is important in React because it makes the code more readable and expressive, allowing developers to write components that look like HTML. JSX is then transpiled into regular JavaScript by tools like Babel. This seamless blend of JavaScript and HTML simplifies the creation and management of UI components.
4. Can you explain the concept of the Virtual DOM in React and its advantages?
The Virtual DOM is a concept where a virtual representation of the UI is kept in memory and synced with the real DOM by a library such as ReactDOM. This process is called reconciliation. The Virtual DOM improves performance by minimizing the number of direct manipulations of the actual DOM, which are costly operations. By making updates in the virtual representation first and then applying the necessary changes to the real DOM in an efficient manner, React can ensure faster and more responsive applications.
5. How do you manage state in a React application?
State in React can be managed using various approaches depending on the complexity of the application. For local component state, the useState hook (or setState in class components) is commonly used. For more complex state management, especially when dealing with global state, tools like Redux, Context API, or MobX are used. The useReducer hook is another way to manage state in more complex scenarios within a component.
6. What are props in React and how do you use them?
Props, short for properties, are read-only inputs passed from a parent component to a child component. They allow data and event handlers to be shared between components. Props are used to configure and customize components, enabling them to be reusable and modular. For example, a Button component might receive props like label and onClick to define its text and behavior when clicked.
7. Can you explain the component lifecycle in React?
In React, the component lifecycle refers to the series of methods that are invoked at different stages of a component’s existence. These stages include mounting (when the component is created and inserted into the DOM), updating (when the component’s props or state change), and unmounting (when the component is removed from the DOM). Class components have lifecycle methods such as componentDidMount, componentDidUpdate, and componentWillUnmount. Functional components use hooks like useEffect to handle side effects and mimic these lifecycle methods.
8. How do you handle events in React?
Handling events in React is similar to handling events in plain JavaScript, but with some syntactical differences. React events are named using camelCase, and you pass a function as the event handler, rather than a string. For example, to handle a click event, you would use <button onClick={handleClick}>Click me</button>. Event handlers in React receive a synthetic event object, which wraps the native event and provides consistent behavior across different browsers.
9. What are hooks in React and can you give examples of how you've used them?
Hooks are functions that let you use state and other React features in functional components. The most commonly used hooks are useState for managing state, useEffect for side effects and lifecycle events, and useContext for consuming context. For example, useState is used to add state to a functional component: const [count, setCount] = useState(0);. useEffect can be used to perform a side effect like fetching data: useEffect(() => { fetchData(); }, []);.
10. Can you explain the difference between a class component and a functional component in React?
Class components are ES6 classes that extend React.Component and include a render method to return JSX. They can have state and lifecycle methods. Functional components, introduced earlier, were stateless but now can use hooks to manage state and lifecycle events, making them more powerful and versatile. Functional components are typically simpler and easier to test and understand.
11. How do you implement forms in React?
Implementing forms in React involves managing the state of the form inputs and handling form submission. You can use controlled components where the form data is handled by the state within the component. For example, you might have const [value, setValue] = useState(''); and an input element with value={value} and onChange={(e) => setValue(e.target.value)}. Handling form submission usually involves an onSubmit event handler that processes the form data.
12. What is Redux and how does it relate to React?
Redux is a state management library often used with React to manage the application’s state in a predictable way. It centralizes the state in a single store, making it easier to manage and debug. Redux uses actions to describe state changes and reducers to handle these actions and update the state. React components can connect to the Redux store using the connect function or hooks like useSelector and useDispatch.
13. Can you discuss a project where you used React and faced challenges?
In a recent project, we used React to build a complex dashboard application. One of the challenges was managing state across many components and ensuring that updates were efficiently propagated. We addressed this by implementing Redux for state management, which helped to centralize state logic and make it easier to debug. We also optimized performance by using React.memo and the useCallback hook to prevent unnecessary re-renders.
14. How do you optimize performance in a React application?
Optimizing performance in a React application can involve several strategies, such as memoizing components with React.memo, using the useMemo and useCallback hooks to avoid unnecessary re-renders, implementing code-splitting with React.lazy and Suspense, and optimizing the rendering process with virtualization techniques using libraries like react-window. Additionally, using efficient data structures and algorithms, and minimizing unnecessary DOM manipulations, can significantly improve performance.
15. What is Context in React and how have you used it?
Context in React is a way to pass data through the component tree without having to pass props down manually at every level. It’s useful for global data that many components need, such as themes or user information. You create a context with React.createContext and use Provider to pass the value down. Components can consume the context using the useContext hook or the Consumer component. For example, I have used Context to manage user authentication state across an application.
16. Can you explain error boundaries in React?
Error boundaries are React components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of the component tree that crashed. Error boundaries are created by implementing either componentDidCatch and getDerivedStateFromError lifecycle methods in a class component. Functional components can’t directly act as error boundaries, but you can use the useErrorBoundary hook from third-party libraries to achieve similar functionality.
17. How do you handle routing in a React application?
Routing in a React application is typically handled using libraries like React Router. React Router allows you to define routes and navigate between different components based on the URL. You can use components like BrowserRouter, Route, Switch, and Link to set up client-side routing. For example, <Route path="/home" component={Home} /> defines a route that renders the Home component when the URL path is /home.
18. What are higher-order components in React?
Higher-order components (HOCs) are functions that take a component and return a new component with additional props or behavior. They are used to reuse component logic, such as authentication checks or data fetching, across multiple components. An example of an HOC is a withAuth HOC that checks if a user is authenticated and redirects to a login page if they are not. You create an HOC by wrapping the input component and enhancing it with new functionality.
19. Can you explain how to implement server-side rendering with React?
Server-side rendering (SSR) with React can be implemented using frameworks like Next.js or by setting up your own server with Node.js and Express. SSR involves rendering React components on the server and sending the HTML to the client. This can improve performance and SEO. In Next.js, you can use functions like getServerSideProps to fetch data and render pages on the server. In a custom setup, you can use ReactDOMServer.renderToString to generate HTML from React components on the server.
20. What is React Fiber and why is it important?
React Fiber is the reimplementation of React’s core algorithm for rendering and updating the UI. Introduced in React 16, Fiber improves the ability to split rendering work into chunks and prioritize updates, making React more efficient and responsive. It allows for features like time slicing and suspense, enabling smoother user experiences and better handling of complex animations and interactions.
21. How do you manage global state in React without using external libraries?
Global state in React can be managed without external libraries using the Context API combined with the useReducer hook. The Context API allows you to create a global context and a provider component that supplies state and dispatch functions. useReducer provides a way to handle complex state logic with reducers, similar to Redux. This combination offers a lightweight solution for managing global state in smaller applications or when you want to avoid adding extra dependencies.
22. Can you discuss the differences between controlled and uncontrolled components in React?
Controlled components in React have their state managed by the React component itself. The component's state serves as the single source of truth, and any changes are handled by updating the state through event handlers. For example, an input element with its value controlled by a state variable is a controlled component. Uncontrolled components, on the other hand, rely on the DOM to manage their state. They use refs to access the DOM elements directly and retrieve their values. Controlled components provide better control and synchronization with React state, while uncontrolled components are simpler but less flexible.
23. How do you use React with TypeScript?
Using React with TypeScript involves setting up a TypeScript environment and creating components with type annotations. TypeScript provides static typing, which helps catch errors early and improves code maintainability. You define prop types, state types, and context types to ensure type safety. For example, you can define a component’s props using an interface: interface Props { name: string; age: number; } and then use it in the component: const MyComponent: React.FC<Props> = ({ name, age }) => { ... }.
24. What are some strategies for testing React components?
Testing React components can be done using tools like Jest and React Testing Library. Jest provides a robust testing framework for unit tests, mocks, and assertions. React Testing Library focuses on testing the component’s behavior from the user’s perspective rather than implementation details. Strategies include writing unit tests for individual components, integration tests for component interactions, and snapshot tests to capture the component's output. Ensuring good test coverage and writing tests that reflect real user interactions are key strategies for effective testing.
25. How do you ensure accessibility in a React application?
Ensuring accessibility in a React application involves following best practices and using tools to check for compliance with accessibility standards. Best practices include using semantic HTML, providing meaningful alt text for images, ensuring keyboard navigability, and using ARIA (Accessible Rich Internet Applications) attributes when necessary. Tools like axe-core and Lighthouse can be integrated to automatically detect accessibility issues. Additionally, manual testing with screen readers and other assistive technologies helps ensure a comprehensive accessibility check.
26. What tools do you use for debugging React applications?
For debugging React applications, common tools include the React Developer Tools browser extension, which provides an interface to inspect React component hierarchies, props, and state. Additionally, the browser’s built-in developer tools are useful for debugging JavaScript, inspecting network requests, and examining the DOM. Console logging and breakpoints are fundamental debugging techniques, and tools like Redux DevTools help in debugging state management issues when using Redux.
27. Can you explain the concept of lifting state up in React?
Lifting state up in React involves moving state to a common ancestor component so that it can be shared between sibling components. This pattern is used when multiple components need to share or synchronize state. Instead of maintaining separate states, which can lead to inconsistencies, the state is managed at a higher level in the component hierarchy. The common ancestor then passes the state and state-updating functions down to the child components via props.
28. What is React Portal and when would you use it?
React Portal is a feature that allows you to render a component's subtree outside of its parent DOM hierarchy. It is useful for cases where you need to break out of the typical parent-child DOM structure, such as rendering modals, tooltips, or dropdowns that need to appear above other elements in the DOM. You create a portal using ReactDOM.createPortal(child, container), where child is the component to render and container is the DOM node where it should be rendered.
29. How do you use React in combination with other libraries or frameworks?
React can be used in combination with various libraries and frameworks to enhance its functionality. For state management, libraries like Redux, MobX, or Recoil can be integrated. For routing, React Router is commonly used. To handle form validation, libraries like Formik or React Hook Form are useful. For styling, you can use CSS-in-JS libraries like styled-components or Emotion. React’s flexibility allows it to work seamlessly with other tools and libraries to build comprehensive applications.
30. What are fragments in React and why are they useful?
Fragments in React are a way to group multiple elements without adding extra nodes to the DOM. They are useful when a component needs to return multiple children, but adding an unnecessary wrapper element could affect the styling or structure. Fragments can be created using the <React.Fragment> element or the shorthand <> </>. For example, <><Child1 /><Child2 /></> returns both Child1 and Child2 without any additional DOM nodes.
31. How do you handle animations in React?
Animations in React can be handled using CSS transitions and animations, JavaScript libraries like GSAP, or React-specific libraries like React Transition Group or Framer Motion. CSS transitions and animations are straightforward for simple animations. React Transition Group provides more control over animations, especially for component enter and exit transitions. Framer Motion offers a powerful and easy-to-use API for more complex animations and interactions.
32. Can you explain the significance of keys in React lists?
Keys in React lists are crucial for identifying which items have changed, been added, or removed. They help React optimize the rendering process by minimizing re-renders and improving performance. Each item in a list should have a unique key, typically derived from a unique identifier such as an ID. Using keys ensures that React can efficiently manage and update the list items without unnecessarily re-rendering unchanged components.
33. What is the useMemo hook and how do you use it?
The useMemo hook in React is used to memoize a value, ensuring that it is only recomputed when one of its dependencies changes. This is useful for optimizing performance, especially when dealing with expensive calculations or functions. For example, const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]); will only recompute memoizedValue when a or b changes. This prevents unnecessary recalculations and improves rendering efficiency.
34. How do you implement lazy loading in React?
Lazy loading in React can be implemented using the React.lazy function and the Suspense component. This allows components to be loaded on demand, reducing the initial load time and improving performance. For example, you can create a lazily-loaded component with const LazyComponent = React.lazy(() => import('./LazyComponent')); and then use it with Suspense: <Suspense fallback={<div>Loading...</div>}><LazyComponent /></Suspense>. This will only load LazyComponent when it is needed.
35. What are the common pitfalls you've encountered in React development and how do you avoid them?
Common pitfalls in React development include improper state management leading to performance issues, overusing component re-renders, and not optimizing for large applications. To avoid these, it’s essential to structure state management properly using tools like Redux or Context API, memoize components and functions using React.memo and useCallback, and split the codebase for better performance. Ensuring good practices, like using unique keys in lists and cleaning up side effects in useEffect, also helps prevent issues.
36. How do you use the useContext hook in React?
The useContext hook in React is used to consume context values in functional components. It simplifies the process of accessing global data provided by a context. First, you create a context with const MyContext = React.createContext(defaultValue);. Then, you use a provider to pass the context value down the component tree: <MyContext.Provider value={value}>...</MyContext.Provider>. In a child component, you can consume the context value with const contextValue = useContext(MyContext);.
37. What is React's Concurrent Mode and how does it improve application performance?
React's Concurrent Mode is an experimental feature that improves the responsiveness of applications by allowing React to work on multiple tasks simultaneously. It enables React to interrupt and prioritize rendering tasks, leading to smoother user interactions and faster updates. For example, React can pause rendering lower-priority updates to respond to user input quickly. This improves performance, especially in complex applications with heavy rendering requirements.
38. How do you handle internationalization in React applications?
Internationalization (i18n) in React applications can be handled using libraries like react-intl, i18next, or LinguiJS. These libraries provide tools for managing translations, formatting dates and numbers, and handling different locales. You set up your translations in JSON files and use context providers or hooks to access the translations in your components. For example, with react-intl, you wrap your application with IntlProvider and use the FormattedMessage component or useIntl hook to display translated text.
39. Can you explain the process of migrating a legacy application to React?
Migrating a legacy application to React involves several steps. First, you assess the existing codebase to identify the core components and structure. Next, you set up a new React project and gradually migrate parts of the application, starting with isolated components. You can use techniques like iframes or micro-frontends to integrate React components into the legacy application. It’s important to maintain functionality and test extensively during the migration to ensure a smooth transition.
40. What are PropTypes in React and how are they used?
PropTypes in React are a way to type-check the props passed to components, ensuring they receive the correct data types and values. PropTypes are used by defining a propTypes property on a component. For example, MyComponent.propTypes = { name: PropTypes.string.isRequired, age: PropTypes.number }; specifies that the name prop must be a string and is required, while the age prop should be a number. PropTypes help catch bugs and ensure the correct usage of components.
41. How do you manage environment variables in a React project?
Environment variables in a React project are managed using a .env file. You define variables in this file with a REACT_APP_ prefix, such as REACT_APP_API_URL=https://api.example.com. These variables can then be accessed in the code using process.env.REACT_APP_API_URL. It’s important to avoid exposing sensitive information in the .env file, and tools like dotenv-webpack can be used to manage and bundle environment variables securely.
42. What is the useReducer hook and how does it compare to useState?
The useReducer hook in React is used for managing complex state logic in a component, similar to how Redux manages state. It is an alternative to useState when state transitions are more complex or involve multiple actions. useReducer takes a reducer function and an initial state, and returns the current state and a dispatch function. For example, const [state, dispatch] = useReducer(reducer, initialState);. While useState is simpler and more suitable for basic state, useReducer provides a more structured approach for complex state logic.
43. Can you explain the concept of code splitting in React and how you implement it?
Code splitting in React is a technique used to split the application code into smaller bundles, which are loaded on demand. This reduces the initial load time and improves performance. Code splitting can be implemented using dynamic imports with React.lazy and Suspense, or using tools like Webpack for more advanced configurations. For example, const LazyComponent = React.lazy(() => import('./LazyComponent')); loads the component only when needed, and Suspense provides a fallback UI while loading.
44. What is a pure component in React and how does it differ from a regular component?
A pure component in React is a component that performs a shallow comparison of its props and state to determine if it should re-render. Pure components extend React.PureComponent instead of React.Component. This optimization helps prevent unnecessary re-renders, improving performance. For example, if a component receives the same props and state, a pure component will skip rendering, whereas a regular component would re-render regardless. Pure components are useful when the component’s rendering logic is simple and the props and state are immutable.
45. How do you secure a React application?
Securing a React application involves several practices. First, ensure data validation and sanitization to prevent injection attacks. Use HTTPS to encrypt data in transit and secure cookies with the HttpOnly and Secure flags. Implement authentication and authorization using libraries like OAuth or JWT. Avoid exposing sensitive information in the frontend code and environment variables. Additionally, regularly update dependencies to patch security vulnerabilities and use tools like Helmet to set secure HTTP headers.
46. What are some best practices for structuring a large React codebase?
Best practices for structuring a large React codebase include organizing components into feature-based or domain-based directories, using a consistent naming convention, and separating concerns by grouping related files (e.g., components, styles, tests). Utilize a clear folder hierarchy and keep the root directory clean. Use index files for easier imports and maintain modularity by splitting large components into smaller, reusable pieces. Adopting a consistent style guide and code formatting tools like Prettier and ESLint also helps maintain code quality.
47. How do you handle date and time manipulation in React applications?
Handling date and time manipulation in React applications can be done using libraries like date-fns, Moment.js, or the native JavaScript Date object. date-fns and Moment.js provide extensive functions for parsing, formatting, and manipulating dates and times. For example, format(new Date(), 'MM/dd/yyyy') formats the current date using date-fns. These libraries help simplify complex date operations, ensuring accurate and consistent handling of date and time across the application.
48. What are the benefits of using React Native for mobile application development?
React Native allows developers to build mobile applications using React and JavaScript, enabling code reuse across iOS and Android platforms. This reduces development time and costs. React Native provides a near-native performance and user experience, as it uses native components. The hot-reloading feature improves development speed by instantly reflecting changes. Additionally, a large community and ecosystem offer a wealth of libraries and tools, enhancing development capabilities and support.
49. How do you stay updated with the latest developments and best practices in React?
Staying updated with the latest developments and best practices in React involves following official React documentation, subscribing to React newsletters, and participating in the React community through forums, blogs, and social media. Attending conferences, webinars, and meetups provides insights into new trends and techniques. Additionally, contributing to open-source projects and exploring new libraries and tools helps maintain current knowledge and skills in React development.
50. Can you discuss a time when you significantly improved the performance or user experience of a React application?
In a recent project, I significantly improved the performance and user experience of a React application by implementing code splitting and lazy loading for large components. I used React.lazy and Suspense to load components on demand, reducing the initial load time. Additionally, I optimized state management by using memoization techniques like React.memo and useCallback to prevent unnecessary re-renders. These optimizations resulted in a faster, more responsive application, enhancing the overall user experience and satisfaction.